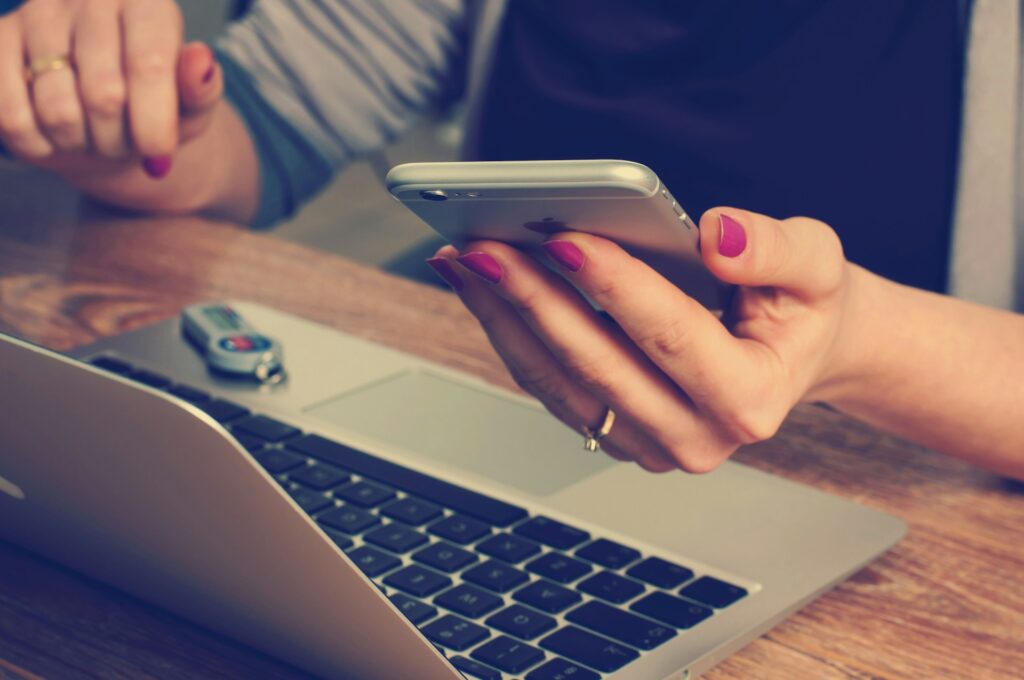
Mobile apps need testing across devices for proper functionality. Appium automates tests for Android and iOS platforms. This Appium tutorial will explain how to set it up and use it effectively for testing.
It allows testing for both Android and iOS apps using a single framework. It supports all types of apps without needing changes to the code. This tutorial is great for beginners or anyone looking to improve their mobile testing skills with clear and practical steps.
You will learn how to set up Appium, create and run your first test script on an Android device, and use frameworks like TestNG or JUnit to organize tests. You will be ready to automate mobile testing and build apps that perform well on different devices by the end of this Appium tutorial.
Mobile Automation Framework – Appium
A mobile automation framework helps test mobile apps effectively and makes sure they work on different devices. It gives a clear way to automate test cases for all kinds of apps. Appium is one of the most popular tools for this.
Appium allows testing on both Android and iOS platforms. It works with different programming languages and platforms making it easy to use and flexible.
Writing Test Scripts in Java for Android Testing
Here is the procedure to write test scripts in Java for Android Testing:
Set Up Your Integrated Development Environment
- Download and Install: Use IDEs like Eclipse or IntelliJ IDEA which are popular choices for Java development.
- Create a New Project: Start a new Java project in your chosen IDE and name it appropriately for your testing project.
- Add Appium and Selenium Dependencies: Use tools like Maven or Gradle to include Appium and Selenium libraries in your project for handling UI automation.
- Set Up a TestNG or JUnit Framework: Install and integrate TestNG or JUnit with your project to streamline test case management.
Import Required Libraries
- Import Appium Libraries: Include essential libraries such as io.appium.java_client.* for mobile-specific functionalities.
- Add Selenium Libraries: Import Selenium libraries to interact with UI elements like buttons, text fields, and drop-down menus.
- Add Other Utility Libraries: Include libraries like java.util or org.openqa.selenium for managing lists, exceptions, and browser interactions.
Define Test Steps
- Create a Base Class: Write a base class to initialize WebDriver and define reusable methods.
- Locate UI Elements: Use locators like ID, XPath, or Accessibility ID to find mobile elements.
- Write Interaction Methods: Define methods to interact with UI components such as clicking a button or entering text into a field.
- Add Assertions: Use assertions to validate the expected outcomes of your test cases.
Setting Desired Capabilities in Appium for Android Devices
Desired capabilities are essential for configuring the Appium server to understand your test environment and target devices.
Define Device Information
- Platform Name: Specify the platform you are targeting such as “Android”.
- Device Name: Provide the device name or emulator name.
- Platform Version: Specify the Android OS version.
Specify App Path
- Absolute App Path: Use the full path to the APK file of the application under test.
- Ensure Compatibility: Confirm that the file is compatible with the Android version you are testing on.
Include Automation Name
- Select Automation Framework: Use “UIAutomator2” for Android as it is more reliable and efficient.
- Avoid Deprecated Frameworks: Do not use older frameworks like “UIAutomator” unless required for legacy apps.
Additional Configurations
- Session Timeout: Specify the maximum time for sessions to remain idle before timeout.
- Full Reset Option: Use fullReset to reinstall the app before every test execution.
- No Reset Option: Use noReset to keep the app state unchanged between tests.
Appium Tutorial to Run and Debug Your First Test on an Android Device
Executing and debugging your first test script is crucial to validate the setup and ensure smooth test execution.
Start the Appium Server
- Launch Appium Server: Open the Appium Desktop application or run the server from the command line using the appium command.
- Check Server Logs: Monitor them to ensure it is running without errors.
Execute the Test Script
- Run from IDE: Use your IDE’s Run option to execute the test script and observe the execution flow.
- Monitor Device Behavior: Watch the emulator or real device to verify that test steps are being performed as expected.
- Handle Initial Errors: Address common setup errors such as incorrect desired capabilities or device connection issues.
Analyze Debug Logs
- Enable Appium Logs: Use verbose logging in Appium to capture detailed logs of the test execution.
- Check Stack Traces: Review stack traces to pinpoint issues in your test script or application.
- Use Breakpoints in IDE: Set breakpoints in your code to pause execution and inspect variables during runtime.
Appium Tutorial with Testing Frameworks Like TestNG and JUnit
Integrating Appium with frameworks like TestNG and JUnit simplifies test management and improves efficiency.
Organize Test Classes
- Separate Test Modules: Group related test cases into separate classes for better readability.
- Create a Base Test Class: Include setup and teardown methods that can be reused across multiple test classes.
- Use Descriptive Class Names: Name classes to indicate the functionality they test, such as LoginTests or SignupTests.
Define Annotations
- Setup Annotations: Use @BeforeTest to initialize WebDriver and set up desired capabilities.
- Teardown Annotations: Use @AfterTest to clean up and close the session after test execution.
- Test Annotations: Use @Test to define individual test cases and include assertions for validation.
Generate Reports
- Use Built-In Reporting: Both TestNG and JUnit offer reporting tools that display test results in a structured format.
- Customize Reports: Use external libraries like ExtentReports for detailed reports with screenshots and logs.
- Analyze Results: Review the reports to identify failed tests and debug issues quickly.
Key Features and Capabilities of Appium for Mobile Automation
Appium offers various features that enhance the mobile automation experience.
Cross-Platform Mobile Testing
- One Script for All Platforms: Test scripts written in Appium work on both Android and iOS devices without modification.
- Reduced Testing Time: Unified scripts save time by eliminating the need for platform-specific test creation.
- Wide Compatibility: Appium supports a variety of device types and operating systems for comprehensive testing.
Appium’s Support for Multiple Programming Languages
- Java Support: Ideal for teams familiar with Java and its rich ecosystem of testing frameworks.
- Python Support: Provides an option for writing clean and concise test scripts for mobile applications.
- JavaScript Support: Allows integration with web development frameworks for a seamless experience.
Real Device and Emulator Support for Testing
- Real Device Testing: Ensures that applications perform as expected under real-world conditions.
- Emulator Testing: Simulates different device configurations for early-stage testing of applications.
- Flexible Switching Between Devices: Allows testers to run the same scripts on both real devices and emulators.
Appium Server Interactions
- Efficient Command Execution: Translates test commands into actions performed on the mobile device.
- Detailed Log Management: Appium generates comprehensive logs to help testers debug issues effectively.
- Parallel Testing Support: Allows running tests on multiple devices simultaneously to save time.
Best Practices for Writing and Managing Appium Test Scripts
Following the practices mentioned below in the Appium tutorial ensures that your test scripts are efficient.
Structuring Tests for Better Readability and Maintenance
- Organize Tests Logically: Group related test scripts into classes and methods to enhance clarity.
- Use Clear Names for Methods: Give methods descriptive names that reflect their purpose for better readability.
- Keep Tests Focused: Ensure each test script focuses on a single functionality for easier debugging.
Using Page Object Model for Scalable Test Automation
- Create Page Classes for Each Screen: Store UI element locators and methods in dedicated page classes.
- Reuse Page Methods Across Tests: Call page methods in different test scripts to avoid redundancy.
- Simplify Maintenance: Update UI element locators in a single place when changes occur in the application.
Handling Synchronization with Implicit and Explicit Waits
- Set Default Wait Times Globally: Use implicit waits to handle minor delays across the application.
- Wait for Specific Conditions: Use explicit waits to pause execution until an element meets predefined criteria.
- Avoid Fixed Delays: Replace static delays with dynamic waits to optimize test execution time.
Managing Test Data Separately for Flexible Test Scenarios
- Store Test Data Externally: Use JSON, Excel, or CSV files to manage test data outside the script.
- Encrypt Sensitive Data: Protect sensitive information like credentials with encryption techniques.
- Use Parameterized Tests: Pass different data sets to the same test script to validate multiple scenarios.
Utilizing Cloud Testing Platforms
- Access to Multiple Devices: Cloud platforms give you access to a wide range of devices. This allows you to perform different types of testing, like AI testing on your app on different models without owning them.
- Scalability for Parallel Testing: Cloud testing lets you run tests on multiple devices at once. This speeds up testing and helps you get results faster.
- Cost-Effective Testing: Cloud testing platforms reduce the need for physical devices and infrastructure.
LambdaTest is an AI-powered testing platform that assists in conducting both manual and automated tests. You can conduct tests on more than 3000 actual devices, browsers, and operating systems. It employs a cloud-based infrastructure for conducting tests. This enables you to test the performance of the app on actual devices. The findings are precise and dependable.
LambdaTest is compatible with tools such as Appium, Espresso, and XCUITest. These tools speed up and simplify the testing process.
IContinuous Integration and Test Automation
- Connect Appium with continuous integration tools like Jenkins. This allows your tests to run automatically every time you make changes to the code.
- Set up tests to run automatically on code commits. This helps identify issues early and ensures that your code is always working correctly.
Conclusion
Appium is a tool that makes mobile automation easier for Android and iOS. It can test all kinds of apps by allowing testers to use one script for different platforms. Setting up your environment, writing test scripts, and debugging may seem difficult but this Appium tutorial explains each step in a simple way. You can create structured and scalable test cases by integrating Appium with frameworks like TestNG and JUnit.